HogoShi_Kitsune
Legend
Fallout 4 is being released 11/10/15. So, what am I doing to celebrate? Countdown timers!
Web based version: http://www.falloutcounter.com/ (not made by me)
EXE version: Contact me in Skype (Foxtrek_64) for the compiled binary (Windows Only) or....
Because I'm a big fan of the Open Source Initiative, source code!
Preview:
Legal stuff:

This work is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License'.
Web based version: http://www.falloutcounter.com/ (not made by me)
EXE version: Contact me in Skype (Foxtrek_64) for the compiled binary (Windows Only) or....
Because I'm a big fan of the Open Source Initiative, source code!
Form1.cs
form1.designer.cs
This code is automatically generated by Visual Studio
Code:
using System;
using System.Drawing;
using System.Windows.Forms;
namespace Fallout4Countdown
{
public partial class Form1 : Form
{
//Declare the variables that allow you to drag the form about
private bool dragging;
private Point dragAt = Point.Empty;
//This method is called when you have locked onto a control so you can drag the form around
public void Pick(Control control, int x, int y)
{
dragging = true;
dragAt = new Point(x, y);
control.Capture = true;
}
//This method is called when you release the control
public void Drop(Control control)
{
dragging = false;
control.Capture = false;
}
public Form1()
{
//Draw all of the components on the winform
InitializeComponent();
}
//Form1_Load is called when Form1 finishes loading (After InitializeComponent()).
//All it does is start the timer ticking.
private void Form1_Load(object sender, EventArgs e)
{
timer1.Start();
}
//timer1_Tick is called every time a 'tick' event is raised.
//A timer's job is to 'tick' every x ms.
//timer1 ticks every 1000 ms, or once a second.
private void timer1_Tick(object sender, EventArgs e)
{
//Define our two working variables.
//DateTime.Parse(string date) takes a date string with or without time.
//By parsing it, you have it read the string and, based on the local settings of your computer,
//turn that into a date and time.
//
//In this case, it's 11-10-2015 because I'm in the US and we write months first.
//Change to 10-11-2015 for European time.
DateTime releaseDate = DateTime.Parse("11-10-2015");
DateTime timeNow = DateTime.Now;
if (releaseDate > timeNow)
{
//Calculate the difference between the release date and nonw
TimeSpan difference = releaseDate - timeNow;
//Set each of the labels to the correct time
label1.Text = toTime((int)difference.Days);
label2.Text = toTime((int)difference.Hours);
label3.Text = toTime((int)difference.Minutes);
label4.Text = toTime((int)difference.Seconds);
}
else //released
{
object newLine = Environment.NewLine;
label1.Text = "O";
label5.Text = "P";
label2.Text = "E";
label6.Text = "N";
label3.Text = "I";
label7.Text = "N";
label4.Text = "G";
label8.Text = String.Format("PLEASE{0}STAND{0}BACK{0}", newLine);
label9.Text = "VAULT";
label10.Text = "111";
label11.Text = String.Format("PLEASE{0}STAND{0}BACK{0}", newLine);
}
}
//This is where the magic happens
//
//The reason this method exists is two-fold.
//Reason 1: by having one method, you reduce the redundency of the program significantly.
//Reason 2: DateTime lops the leading zero. If you had a clock, it would show up as 12:3 instead of 12:03/
//This method checks to see if that particular block is less than 10. If it is, add a zero. If not, leave it be.
private string toTime(int time)
{
string text;
if (time < 10)
{
text = "0" + time.ToString();
}
else
{
text = time.ToString();
}
return text;
}
//This method is called whenever the mouse button is held down
private void TableLayoutPanel1_MouseDown(object sender, MouseEventArgs e)
{
Pick((Control)sender, e.X, e.Y);
}
//This method is called whenever the mouse button is released
private void TableLayoutPanel1_MouseUp(object sender, MouseEventArgs e)
{
Drop((Control)sender);
}
//This method is called when you move the mouse around
private void TableLayoutPanel1_MouseMove(object sender, MouseEventArgs e)
{
if (dragging)
{
Left = e.X + Left - dragAt.X;
Top = e.Y + Top - dragAt.Y;
}
else dragAt = new Point(e.X, e.Y);
}
//The exit button
private void button1_Click(object sender, EventArgs e)
{
//Close with exit code 0 (non-error)
Environment.Exit(0);
}
}
}
This code is automatically generated by Visual Studio
Code:
namespace Fallout4Countdown
{
partial class Form1
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(Form1));
this.timer1 = new System.Windows.Forms.Timer(this.components);
this.label1 = new System.Windows.Forms.Label();
this.tableLayoutPanel1 = new System.Windows.Forms.TableLayoutPanel();
this.label11 = new System.Windows.Forms.Label();
this.label10 = new System.Windows.Forms.Label();
this.label9 = new System.Windows.Forms.Label();
this.label8 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
this.label5 = new System.Windows.Forms.Label();
this.label6 = new System.Windows.Forms.Label();
this.label7 = new System.Windows.Forms.Label();
this.button1 = new System.Windows.Forms.Button();
this.tableLayoutPanel1.SuspendLayout();
this.SuspendLayout();
//
// timer1
//
this.timer1.Interval = 1000;
this.timer1.Tick += new System.EventHandler(this.timer1_Tick);
//
// label1
//
this.label1.BackColor = System.Drawing.Color.Transparent;
this.label1.Dock = System.Windows.Forms.DockStyle.Fill;
this.label1.Font = new System.Drawing.Font("Microsoft Sans Serif", 48F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label1.ForeColor = System.Drawing.Color.White;
this.label1.Location = new System.Drawing.Point(72, 144);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(105, 105);
this.label1.TabIndex = 1;
this.label1.Text = "11";
this.label1.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// tableLayoutPanel1
//
this.tableLayoutPanel1.AutoSize = true;
this.tableLayoutPanel1.AutoSizeMode = System.Windows.Forms.AutoSizeMode.GrowAndShrink;
this.tableLayoutPanel1.BackColor = System.Drawing.SystemColors.Control;
this.tableLayoutPanel1.BackgroundImage = global::Fallout4Countdown.Properties.Resources.mesh;
this.tableLayoutPanel1.ColumnCount = 9;
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 111F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 111F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 111F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 111F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 111F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 111F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 111F));
this.tableLayoutPanel1.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F));
this.tableLayoutPanel1.Controls.Add(this.label11, 7, 2);
this.tableLayoutPanel1.Controls.Add(this.label10, 5, 2);
this.tableLayoutPanel1.Controls.Add(this.label9, 3, 2);
this.tableLayoutPanel1.Controls.Add(this.label8, 1, 2);
this.tableLayoutPanel1.Controls.Add(this.label1, 1, 1);
this.tableLayoutPanel1.Controls.Add(this.label2, 3, 1);
this.tableLayoutPanel1.Controls.Add(this.label3, 5, 1);
this.tableLayoutPanel1.Controls.Add(this.label4, 7, 1);
this.tableLayoutPanel1.Controls.Add(this.label5, 2, 1);
this.tableLayoutPanel1.Controls.Add(this.label6, 4, 1);
this.tableLayoutPanel1.Controls.Add(this.label7, 6, 1);
this.tableLayoutPanel1.Controls.Add(this.button1, 8, 0);
this.tableLayoutPanel1.Dock = System.Windows.Forms.DockStyle.Fill;
this.tableLayoutPanel1.ForeColor = System.Drawing.SystemColors.ControlText;
this.tableLayoutPanel1.Location = new System.Drawing.Point(0, 0);
this.tableLayoutPanel1.Name = "tableLayoutPanel1";
this.tableLayoutPanel1.RowCount = 4;
this.tableLayoutPanel1.RowStyles.Add(new System.Windows.Forms.RowStyle(System.Windows.Forms.SizeType.Percent, 50F));
this.tableLayoutPanel1.RowStyles.Add(new System.Windows.Forms.RowStyle(System.Windows.Forms.SizeType.Absolute, 105F));
this.tableLayoutPanel1.RowStyles.Add(new System.Windows.Forms.RowStyle(System.Windows.Forms.SizeType.Absolute, 105F));
this.tableLayoutPanel1.RowStyles.Add(new System.Windows.Forms.RowStyle(System.Windows.Forms.SizeType.Percent, 50F));
this.tableLayoutPanel1.Size = new System.Drawing.Size(915, 498);
this.tableLayoutPanel1.TabIndex = 2;
this.tableLayoutPanel1.MouseDown += new System.Windows.Forms.MouseEventHandler(this.TableLayoutPanel1_MouseDown);
this.tableLayoutPanel1.MouseMove += new System.Windows.Forms.MouseEventHandler(this.TableLayoutPanel1_MouseMove);
this.tableLayoutPanel1.MouseUp += new System.Windows.Forms.MouseEventHandler(this.TableLayoutPanel1_MouseUp);
//
// label11
//
this.label11.BackColor = System.Drawing.Color.Transparent;
this.label11.Dock = System.Windows.Forms.DockStyle.Fill;
this.label11.Font = new System.Drawing.Font("Microsoft Sans Serif", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label11.ForeColor = System.Drawing.Color.White;
this.label11.Location = new System.Drawing.Point(738, 249);
this.label11.Name = "label11";
this.label11.Size = new System.Drawing.Size(105, 105);
this.label11.TabIndex = 11;
this.label11.Text = "SECONDS";
this.label11.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label10
//
this.label10.BackColor = System.Drawing.Color.Transparent;
this.label10.Dock = System.Windows.Forms.DockStyle.Fill;
this.label10.Font = new System.Drawing.Font("Microsoft Sans Serif", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label10.ForeColor = System.Drawing.Color.White;
this.label10.Location = new System.Drawing.Point(516, 249);
this.label10.Name = "label10";
this.label10.Size = new System.Drawing.Size(105, 105);
this.label10.TabIndex = 10;
this.label10.Text = "MINUTES";
this.label10.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label9
//
this.label9.BackColor = System.Drawing.Color.Transparent;
this.label9.Dock = System.Windows.Forms.DockStyle.Fill;
this.label9.Font = new System.Drawing.Font("Microsoft Sans Serif", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label9.ForeColor = System.Drawing.Color.White;
this.label9.Location = new System.Drawing.Point(294, 249);
this.label9.Name = "label9";
this.label9.Size = new System.Drawing.Size(105, 105);
this.label9.TabIndex = 9;
this.label9.Text = "HOURS";
this.label9.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label8
//
this.label8.BackColor = System.Drawing.Color.Transparent;
this.label8.Dock = System.Windows.Forms.DockStyle.Fill;
this.label8.Font = new System.Drawing.Font("Microsoft Sans Serif", 14.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label8.ForeColor = System.Drawing.Color.White;
this.label8.Location = new System.Drawing.Point(72, 249);
this.label8.Name = "label8";
this.label8.Size = new System.Drawing.Size(105, 105);
this.label8.TabIndex = 8;
this.label8.Text = "DAYS";
this.label8.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label2
//
this.label2.BackColor = System.Drawing.Color.Transparent;
this.label2.Dock = System.Windows.Forms.DockStyle.Fill;
this.label2.Font = new System.Drawing.Font("Microsoft Sans Serif", 48F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label2.ForeColor = System.Drawing.Color.White;
this.label2.Location = new System.Drawing.Point(294, 144);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(105, 105);
this.label2.TabIndex = 2;
this.label2.Text = "11";
this.label2.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label3
//
this.label3.BackColor = System.Drawing.Color.Transparent;
this.label3.Dock = System.Windows.Forms.DockStyle.Fill;
this.label3.Font = new System.Drawing.Font("Microsoft Sans Serif", 48F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label3.ForeColor = System.Drawing.Color.White;
this.label3.Location = new System.Drawing.Point(516, 144);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(105, 105);
this.label3.TabIndex = 3;
this.label3.Text = "11";
this.label3.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label4
//
this.label4.BackColor = System.Drawing.Color.Transparent;
this.label4.Dock = System.Windows.Forms.DockStyle.Fill;
this.label4.Font = new System.Drawing.Font("Microsoft Sans Serif", 48F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label4.ForeColor = System.Drawing.Color.White;
this.label4.Location = new System.Drawing.Point(738, 144);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(105, 105);
this.label4.TabIndex = 4;
this.label4.Text = "11";
this.label4.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label5
//
this.label5.BackColor = System.Drawing.Color.Transparent;
this.label5.Dock = System.Windows.Forms.DockStyle.Fill;
this.label5.Font = new System.Drawing.Font("Microsoft Sans Serif", 48F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label5.ForeColor = System.Drawing.Color.White;
this.label5.Location = new System.Drawing.Point(183, 144);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(105, 105);
this.label5.TabIndex = 5;
this.label5.Text = "|";
this.label5.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label6
//
this.label6.BackColor = System.Drawing.Color.Transparent;
this.label6.Dock = System.Windows.Forms.DockStyle.Fill;
this.label6.Font = new System.Drawing.Font("Microsoft Sans Serif", 48F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label6.ForeColor = System.Drawing.Color.White;
this.label6.Location = new System.Drawing.Point(405, 144);
this.label6.Name = "label6";
this.label6.Size = new System.Drawing.Size(105, 105);
this.label6.TabIndex = 6;
this.label6.Text = "|";
this.label6.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// label7
//
this.label7.BackColor = System.Drawing.Color.Transparent;
this.label7.Dock = System.Windows.Forms.DockStyle.Fill;
this.label7.Font = new System.Drawing.Font("Microsoft Sans Serif", 48F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.label7.ForeColor = System.Drawing.Color.White;
this.label7.Location = new System.Drawing.Point(627, 144);
this.label7.Name = "label7";
this.label7.Size = new System.Drawing.Size(105, 105);
this.label7.TabIndex = 7;
this.label7.Text = "|";
this.label7.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// button1
//
this.button1.AutoSize = true;
this.button1.BackColor = System.Drawing.Color.Transparent;
this.button1.FlatAppearance.BorderColor = System.Drawing.Color.Silver;
this.button1.FlatAppearance.BorderSize = 2;
this.button1.FlatStyle = System.Windows.Forms.FlatStyle.Flat;
this.button1.Font = new System.Drawing.Font("Consolas", 9.75F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.button1.ForeColor = System.Drawing.Color.Silver;
this.button1.Location = new System.Drawing.Point(849, 3);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(63, 29);
this.button1.TabIndex = 12;
this.button1.Text = "[EXIT]";
this.button1.UseVisualStyleBackColor = false;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(915, 498);
this.Controls.Add(this.tableLayoutPanel1);
this.ForeColor = System.Drawing.Color.Silver;
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.None;
this.Icon = ((System.Drawing.Icon)(resources.GetObject("$this.Icon")));
this.MaximizeBox = false;
this.Name = "Form1";
this.Text = "Fallout Countdown";
this.TransparencyKey = System.Drawing.SystemColors.Control;
this.Load += new System.EventHandler(this.Form1_Load);
this.tableLayoutPanel1.ResumeLayout(false);
this.tableLayoutPanel1.PerformLayout();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Timer timer1;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TableLayoutPanel tableLayoutPanel1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Label label5;
private System.Windows.Forms.Label label6;
private System.Windows.Forms.Label label7;
private System.Windows.Forms.Label label11;
private System.Windows.Forms.Label label10;
private System.Windows.Forms.Label label9;
private System.Windows.Forms.Label label8;
private System.Windows.Forms.Button button1;
}
}
Preview:
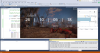
Legal stuff:

This work is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License'.
Last edited: